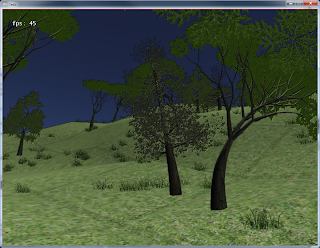
In my original code, way back when I was basically working through
Riemer Grootjan's excellent tutorials, I stumbled upon
LTrees, a procedural tree generation system that worked with XNA. It was really quite straightforward, and I easily added it in.
My current voxel implementation is more complicated however, and adding the intricacies of deferred rendering (I swear I will eventually get around to discussing Project Vanquish and what I've been doing with it), I'd put off re-integrating it for a while.
I've finally done that, and it was
mostly easy. Generally speaking I had to change the shaders to deal with deferred rendering, adding normal and depth to the pixel shader output.
I've not fiddled with getting shadows to work yet, but I can see my way through that.
There are two other real issues left.
The first is alpha blending on the leaves. Alpha blending is a bit of an Achilles heel for deferred rendering setups. The funny light coloring around the leaves is due to the alpha problems. The second is that... well the naive implementation of simply drawing a lot of trees works slowly, of course.
So, I'm working to implement LTrees, but make the items themselves instanced. That will take a bit of work, so in the meanwhile, these images are what it looks like now.